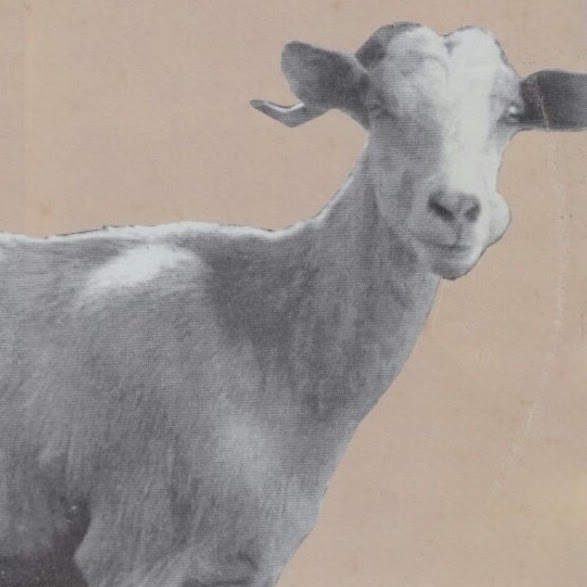
Decided to spent some time and finally ordered an Arduino board and some misc parts. Always wanted to mess around with one, although I really fail to grasp the basic electric stuff. Anyway, here’s what it takes to compile something and upload it on an Arduino board on Gentoo Linux.
There are quite a few guides on the net, and even on Gentoo’s own wiki on how to setup things. I’ve taken some things from all over the place, and with my mind set up not to use the official IDE, here’s what needs to be done:
- Set up the required modules in kernel for USB connection.
Just follow the guide on https://wiki.gentoo.org/wiki/Arduino#Prepare_the_kernel_for_USB_connection to build and install the required modules.
- Setup an avr cross compiler
Luckily, Gentoo makes it really easy to add a cross compiler. So we’ll setup one. First emerge crossdev:
# emerge crossdev
This is just a script which will build libc, gcc and binutils for avr target. Run it:
# USE="-openmp -hardened -sanitize -vtv" crossdev -s4 -S --target avr
and grab a cup of coffee…
After it’s done, run the following to fix some weird linking error (if on amd64):
# ln -s /usr/x86_64-pc-linux-gnu/avr/lib/ldscripts /usr/avr/lib/ldscripts
- Install avrdude & pyserial
This is needed to upload the binary to the arduino. Just:
# emerge avrdude pyserial
- Download the arduino sdk
Update Oct 2020: It seems that Arduino has split it’s repository and removed the sdk header files and libraries from the main Arduino repo. Instead you should checkout the repository of ArduinoCore-avr.
From what I gathered you could actually just emerge arduino which will install everything required. But, remember we don’t want the official IDE!. So just download the arduino stuff for it’s github page. I choose to install everything in /opt/arduino-sdk/
Create a dir as root there and run:
git clone https://github.com/arduino/Arduino.git cd Arduino git checkout 1.6.6
Check the github page for any new release and adjust the checkout accordingly.
- Download a helper Makefile generator and uploader.
This brings everything together, and allows running just make to build an arduino project. In the same directory with the arduino-sdk, run:
git clone https://github.com/sudar/Arduino-Makefile
Now, create a new project somewhere. You’ll need a makefile and the source file.
Makefile:
PROJECT_DIR = /home/evas/stuff/coding/arduino-hello/ ARDMK_DIR = /opt/arduino-sdk/Arduino-Makefile ARCHITECTURE = avr ARDUINO_DIR = /opt/arduino-sdk/Arduino USER_LIB_PATH := $(PROJECT_DIR)/lib BOARD_TAG = uno MONITOR_BAUDRATE = 115200 AVR_TOOLS_DIR = /usr AVRDUDE = /usr/bin/avrdude AVRDUDE_CONF = /etc/avrdude.conf CFLAGS_STD = -std=gnu11 CXXFLAGS_STD = -std=gnu++11 CXXFLAGS += -pedantic -Wall -Wextra MONITOR_PORT = /dev//ttyACM0 CURRENT_DIR = $(shell basename $(CURDIR)) OBJDIR = $(PROJECT_DIR)/bin/$(BOARD_TAG)/$(CURRENT_DIR) include $(ARDMK_DIR)/Arduino.mk
Just edit the above file to suit your needs. A simple test source:
// the setup function runs once when you press reset or power the board void setup() { // initialize digital pin LED_BUILTIN as an output. pinMode(LED_BUILTIN, OUTPUT); } // the loop function runs over and over again forever void loop() { digitalWrite(LED_BUILTIN, HIGH); // turn the LED on (HIGH is the voltage level) delay(1000); // wait for a second digitalWrite(LED_BUILTIN, LOW); // turn the LED off by making the voltage LOW delay(1000); // wait for a second }
Then just run make. If everything went well, it should compile everything up in a bin/uno/arduino-hello/arduino-hello.hex file, ready to be uploaded. To upload to the arduino, just run:
make upload